Week 1 of CS50 introduced a lot of the “ancient” programming language C, alongside how formulas work in general programming. We were to setup a Visual Studio Code Codespace to get ourselves situated with some simple logic “puzzle” programming.
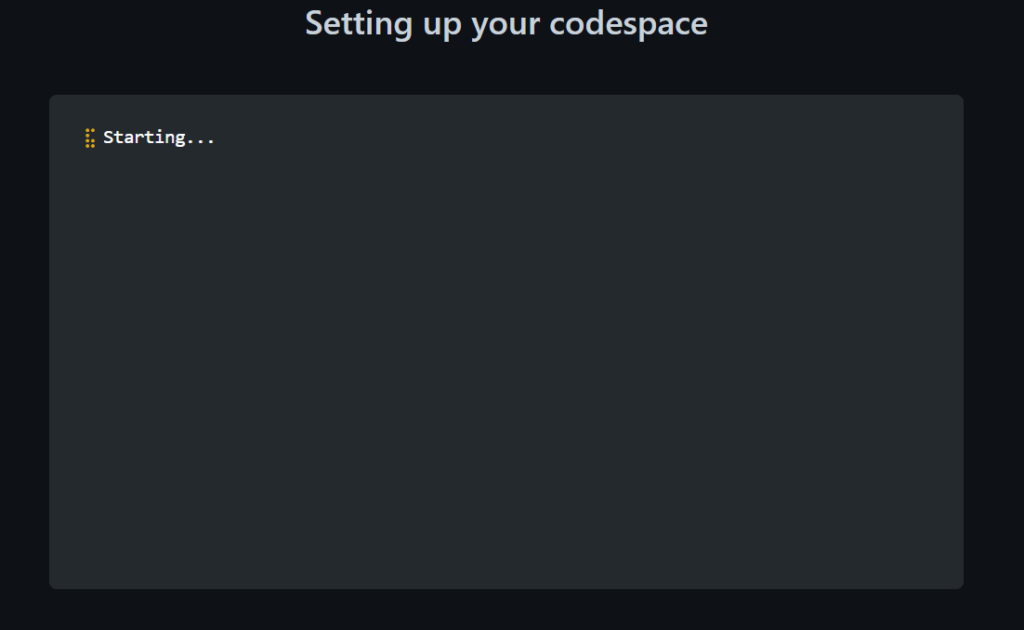
Our first small project, was of course, what you would think: Hello, world, with a twist of a name prompt instead of world.
#include <stdio.h>
#include <cs50.h>
int main(void)
{
// Name request.
string name = get_string("What is your name? ");
// Print hello string with name requested.
printf("Hello, %s\n", name);
}
Followed after by a more elaborate approach. we were to emulate Mario “blocks” using code, resulting essentially in something like the following:
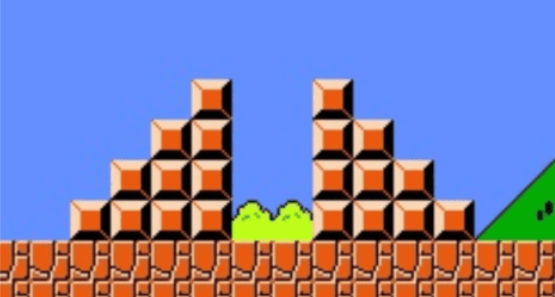
Code was created to generate hashtags based on an input that would create a pyramid like display in the console with the gap included. When broken down multiple steps, it definitely became far easier than to take the whole idea in at once. A decent amount of while loops were used in this scenario.
#include <stdio.h>
#include <cs50.h>
int main(void)
{
int n;
do
{
// Ask for Height
n = get_int("Height: ");
}
// Answer must be between or equal to 1 to 8
while (n < 1 || n > 8);
for (int i = 0; i < n; i++)
{
// Print space, count total height - 1
for (int j = n - 1; j > i; j--)
{
printf(" ");
}
// Print block, amount = to 1++ up to height
for (int k = 0; k <= i; k++)
{
printf("#");
}
// Spacer
printf(" ");
// Print block, without space.
for (int k = 0; k <= i; k++)
{
printf("#");
}
// New line
printf("\n");
}
}
With this code, when a user inputs a value (between 1 and 8 for simplicity), the following is generated in the console. In this case, let’s say 5 is inputted:
Height: 5
# #
## ##
### ###
#### ####
##### #####
A little rudimentary, but this was the intent after all. Using the C language made it not as “quick” as I’m used to as it involved a lot of correctly typing the for loops, regardless of their identical function in JavaScript or most any other language.
In addition to the Hello, World introduction and the Mario project we had a choice between two projects: Cash or Credit. Cash required us to deduce how many coins someone would be holding if they had X amount of cents, X being the amount in coins. Some functions were already created prior and it was up to us to fill them in appropriately. The end result looks, hopefully, self-explanatory:
#include <cs50.h>
#include <stdio.h>
int get_cents(void);
int calculate_quarters(int cents);
int calculate_dimes(int cents);
int calculate_nickels(int cents);
int calculate_pennies(int cents);
int main(void)
{
// Ask how many cents the customer is owed
int cents = get_cents();
// Calculate the number of quarters to give the customer
int quarters = calculate_quarters(cents);
cents = cents - quarters * 25;
// Calculate the number of dimes to give the customer
int dimes = calculate_dimes(cents);
cents = cents - dimes * 10;
// Calculate the number of nickels to give the customer
int nickels = calculate_nickels(cents);
cents = cents - nickels * 5;
// Calculate the number of pennies to give the customer
int pennies = calculate_pennies(cents);
cents = cents - pennies * 1;
// Sum coins
int coins = quarters + dimes + nickels + pennies;
// Print total number of coins to give the customer
printf("%i\n", coins);
}
int get_cents(void)
{
int x;
do
{
x = get_int("Enter the amount of cents: ");
}
while (x < 0);
return x;
}
int calculate_quarters(int cents)
{
int quarterCount;
quarterCount = cents / 25;
return quarterCount;
}
int calculate_dimes(int cents)
{
int dimeCount = cents / 10;
return dimeCount;
}
int calculate_nickels(int cents)
{
int nickCount = cents / 5;
return nickCount;
}
int calculate_pennies(int cents)
{
int penCount = cents / 1;
return penCount;
}
The other option to attempt is one that involves determining whether a credit card is issued by VISA, American Express, or Discover, alongside if the card is a valid card number. For this harder project, we actually were not given any prewritten code on how to start a validation process, but were given information on it. Such as how how the algorithm to determine everything stated above was created by Hans Peter Luhn of IBM, and a detailed explanation of how it works, so that we could translate that information into code.
This proved to be difficult. The concept of validating is initially the entered card was of adequate digits was easy. Followed by checking the prerequisites of what the card begins with and how many digits are present. However, the complex bit was actually implementing the algorithm in C. Luhn’s algorithm, or what is essentially the checksum, takes every other number beginning from the right-most second digit and multiples each by two initially. Then you add those totals together, however if any digits multiplied by two equal a total greater than nine, as in more than one digit, you take each individual digit and add them to the total. Example being if the digit with 6 multiplied by 2, it would equal “12”, so then to the total “1 + 2” is added, not “12”. So after you add these together, you take another step to add the remaining digits in the card, what would have been every other even digits starting from the right. The grand total here, in the end, should be a number divisible by ten. If so, then the credit card number entered is valid! This is the troublesome step.
The explanation above is the meat and heart of the project, the rest is basic repetition of what one should already know. However, for the time being, I am debating attempting this project or moving on to the next week.